Here you will get the program to implement lexical analyzer in C and C++.
The compiler is responsible for converting high-level language into machine language. There are several phases involved in this and lexical analysis is the first phase.
A lexical analyzer reads the characters from the source code and converts them into tokens.
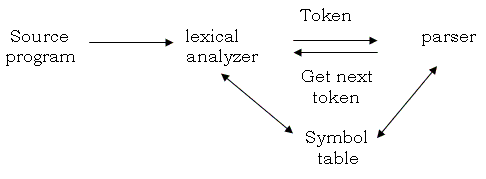
Different tokens or lexemes are:
- Keywords
- Identifiers
- Operators
- Constants
Take the below example.
c = a + b;
After lexical analysis, a symbol table is generated as given below.
Token | Type |
c | identifier |
= | operator |
a | identifier |
+ | operator |
b | identifier |
; | separator |
Now below I have given the implementation of a very simple lexical analyzer that reads source code from a file and then generates tokens.
Program for Lexical Analyzer in C
#include<stdio.h> #include<stdlib.h> #include<string.h> #include<ctype.h> int isKeyword(char buffer[]){ char keywords[32][10] = {"auto","break","case","char","const","continue","default", "do","double","else","enum","extern","float","for","goto", "if","int","long","register","return","short","signed", "sizeof","static","struct","switch","typedef","union", "unsigned","void","volatile","while"}; int i, flag = 0; for(i = 0; i < 32; ++i){ if(strcmp(keywords[i], buffer) == 0){ flag = 1; break; } } return flag; } int main(){ char ch, buffer[15], operators[] = "+-*/%="; FILE *fp; int i,j=0; fp = fopen("program.txt","r"); if(fp == NULL){ printf("error while opening the file\n"); exit(0); } while((ch = fgetc(fp)) != EOF){ for(i = 0; i < 6; ++i){ if(ch == operators[i]) printf("%c is operator\n", ch); } if(isalnum(ch)){ buffer[j++] = ch; } else if((ch == ' ' || ch == '\n') && (j != 0)){ buffer[j] = '\0'; j = 0; if(isKeyword(buffer) == 1) printf("%s is keyword\n", buffer); else printf("%s is indentifier\n", buffer); } } fclose(fp); return 0; }
Program for Lexical Analyzer in C++
#include<iostream> #include<fstream> #include<stdlib.h> #include<string.h> #include<ctype.h> using namespace std; int isKeyword(char buffer[]){ char keywords[32][10] = {"auto","break","case","char","const","continue","default", "do","double","else","enum","extern","float","for","goto", "if","int","long","register","return","short","signed", "sizeof","static","struct","switch","typedef","union", "unsigned","void","volatile","while"}; int i, flag = 0; for(i = 0; i < 32; ++i){ if(strcmp(keywords[i], buffer) == 0){ flag = 1; break; } } return flag; } int main(){ char ch, buffer[15], operators[] = "+-*/%="; ifstream fin("program.txt"); int i,j=0; if(!fin.is_open()){ cout<<"error while opening the file\n"; exit(0); } while(!fin.eof()){ ch = fin.get(); for(i = 0; i < 6; ++i){ if(ch == operators[i]) cout<<ch<<" is operator\n"; } if(isalnum(ch)){ buffer[j++] = ch; } else if((ch == ' ' || ch == '\n') && (j != 0)){ buffer[j] = '\0'; j = 0; if(isKeyword(buffer) == 1) cout<<buffer<<" is keyword\n"; else cout<<buffer<<" is indentifier\n"; } } fin.close(); return 0; }
Output
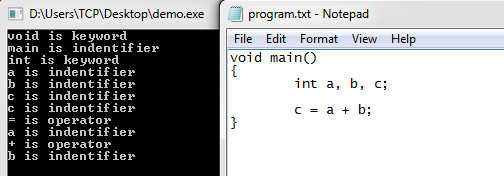
The source code present in the file is shown in the above image.
The below video explains how a lexical analyzer works.
Comment below if you have any queries regarding the above program for lexical analyzer in C and C++.
please explain this program
error while opening a file what can i do
In Turbo c++when i run this program in c++ language it give ma an error like that
‘is_open’ is not a member of ifstream
Bro, its fstream, not ifstream. See if that works.
use devc
While running this program its wont read d contents my file name is 5a.c what can i do??
At the top of the code, in the part of including libraries, has an error, it is written: #include and, in fact, it is: #include
build an text file in the name program.txt with a sample c/c++ programme and compile
before u run the program,u should create a file which saved in the name program.txt.
then u will get the o/p
I created a file with same name and code. Can you please tell where to save this file in order for this code to execute properly.
Create a file called program.txt in the same folder and add some small code in it
lexical Analyzer is mainly used for identifying each and every elements of a program
A file is created in order to check whether the given lexeme is an identifier,keyword or constant.
a function is used to check all the 32 keywords.
geee output ma error while opening the file arha haa
before u run the program,u should create a file which saved in the name program.txt.
then u will get the o/p
I created a file and named it the program.txt yet I receive the same error ” Error while opening the file”
Me also trouble to understand that, so if understand then explain me plzz
The code is running perfectly with no errors, but the output screen does not hold. It vanishes immediately. What should I do please tell me. I’ll be very grateful to you.
which ide or compiler you are using?
Open Command Prompt, go to program location and then run the executable.
Use this code
System(“pause”);
At the very end of the code. Just above the int main() coily bracket.
Int main() {
//ur own code
System(“pause”) ;
}
include —–
getch() at the end of the program in c language
only to be included if conio.h directive is included
use
getch();
at the end
getch();
Use getch() ; at the end of main function
The code is running perfectly with no errors, but the output screen does not hold. It vanishes immediately. What should I do please tell me. I’ll be very grateful to you.
Compiler is responsible for converting high level language in machine language. There are several phases involved in this and lexical analysis is the first phase.std;
int isKeyword(char buffer[]){
char keywords[32][10] = {“auto”,”break”,”case”,”char”,”const”,”continue”,”default”,
“do”,”double”,”else”,”enum”,”extern”,”float”,”for”,”goto”,
“if”,”int”,”long”,”register”,”return”,”short”,”signed”,
“sizeof”,”static”,”struct”,”switch”,”typedef”,”union”,
“unsigned”,”void”,”vola
hola hola hola hooooooooooooo!!!!!!!!!!!!!!!!!!!!!!!!!
ha ha hola hola hola :v
use getch() commad before the last braces
getch();
for C language
while for c++
use
system(“pause”);
if the input is an identifier ‘abc3’, it shows abc is an identifier and 3 is a digit.. please put a program accordingly to identify digits in identifiers and delimiters
in c program write 3 line code to hold the output
#include //after #include
getch(); // last line inside int main()
clrscr(); // next line just after getch();
can you please explain the code. thanks
what should we do for
printf(“hii”);
thank you it was very usefull
but next plz add algorithm to understand code better for non programs
to work easier .
happy day !!!!!
Can you make a lexical for constants and comments
22 Errors o my god??????
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
namespace ConsoleApplication6
{class Program
{static void Main(string[] args)
{string opr = “”;
string num = “”;
string type = “”;
string iden = “”;
String pattern = @”([-+*>/=<])";
string path1 = @"E:input.txt";
string exp = System.IO.File.ReadAllText(path1);
Regex delimiterChars = new Regex(@"[_{;(,.}:)]");
Regex comments1 = new Regex(@"//.*?n");//single line
Regex comments2 = new Regex(@"/*(.|n)*?*/");//multiple line
string afterremoval = comments1.Replace(exp, "");
afterremoval = comments2.Replace(afterremoval, "");
string removal = delimiterChars.Replace(afterremoval, " ");
Regex headerfile = new Regex(@"using.*?n");
Match header = headerfile.Match(removal);
Console.WriteLine("headerfile:{0}", header);
string headerremoval = headerfile.Replace(removal, "");
string[] elements =headerremoval.Split(' ');
foreach (var a in elements)
{if (a.Contains("int") || a.Contains("char") || a.Contains("string") ||
a.Contains("double") || a.Contains("bool") || a.Contains("void") || a.Contains("return") || a.Contains("Main") || a.Contains("static"))
{type = a;
Console.WriteLine("Keyword:{0}", type);}
else
{String[] element = Regex.Split(a, pattern);
foreach (var c in element)
{if (c.Contains("+") || c.Contains("-") || c.Contains("*") || c.Contains("/") || c.Contains("”) || c.Contains(“=”))
{opr = c;
Console.WriteLine(“Operator:{0}”, opr);}
else if (c.Contains(“1”) || c.Contains(“2”) || c.Contains(“3”) || c.Contains(“4”) || c.Contains(“5”)
|| c.Contains(“6”) || c.Contains(“7”) || c.Contains(“8”) || c.Contains(“9”) || c.Contains(“0”))
{num = c;
Console.WriteLine(“Numeric value:{0}”, num);
}
else if (c.Contains(“a”) || c.Contains(“b”) || c.Contains(“c”) || c.Contains(“d”) || c.Contains(“e”)
|| c.Contains(“f”) || c.Contains(“g”) || c.Contains(“h”) || c.Contains(“i”) || c.Contains(“j”)
|| c.Contains(“k”) || c.Contains(“l”) || c.Contains(“m”) || c.Contains(“n”) || c.Contains(“o”)
|| c.Contains(“p”) || c.Contains(“q”) || c.Contains(“r”) || c.Contains(“s”) || c.Contains(“t”)
|| c.Contains(“u”) || c.Contains(“v”) || c.Contains(“w”) || c.Contains(“x”) || c.Contains(“y”)
|| c.Contains(“z”) || c.Contains(“A”) || c.Contains(“B”) || c.Contains(“C”) || c.Contains(“D”)
|| c.Contains(“E”) || c.Contains(“F”) || c.Contains(“G”) || c.Contains(“H”) || c.Contains(“I”)
|| c.Contains(“J”) || c.Contains(“K”) || c.Contains(“L”) || c.Contains(“M”) || c.Contains(“N”)
|| c.Contains(“O”) || c.Contains(“P”) || c.Contains(“Q”) || c.Contains(“R”) || c.Contains(“S”)
|| c.Contains(“T”) || c.Contains(“U”) || c.Contains(“V”) || c.Contains(“W”) || c.Contains(“X”)
|| c.Contains(“Y”) || c.Contains(“Z”))
{iden = c;
Console.WriteLine(“Identifier:{0}”, iden);}}}}
Console.ReadLine();}}}
Hello Sadia, I’m student of UMT, Lhr. I’ve an assignment for the compiler construction using regex. Your code snippet is useful for me and I have a few questions. Will you please be so kind to help me?
Hello! i’ve got a problem in CodeBlocks , when i run a code in order to navigate throught the stack , the compiler is flashing and deos not returns nothing. I read on the some discussions that i might have problem with the compiler , not the cod. I whant to mention that i don’t have any errors and a few warnings. Can anyone give some advice?
Thanks.
Can we run this on simple turbo c?
use getch(); or system(“PAUSE”) at end of the code just above return 0;
It gives operator. But no result for identifier or keywords
The code works perfectly.
I would appreciate help in getting the code to perform a Syntax Analysis on the output of this lexical analysis. I’ve got a project to use YACC tool for it.
Would sincerely appreciate any help.
need syntax analyzer code in c++
in turbo c there is an error on while loop. the error is “while statement is missing”. plzzzz tell me the solution
the programm is running but with message “error while opening the file”. can you fix that ?
This one is work perfectly !! I have checked this one in my lab !! Great job guys !!
it shows all the input as an identifier rather than keywords and operators, and i want to show digits and identifiers separately. what i need to do please help
This program showing all the inputs as an identifier rather than keywords and operators, and i want to show digits and identifiers separately. what i need to do please help.
what is “isalnum” in the line ‘if(isalnum(ch)’ ?
if character is alphabet or number then it will return 1
else return 0
showing “error while opening file”. I’m using codeblocks on macos.
Please help.
else if((ch == ‘ ‘ || ch == ‘\n’) && (j != 0)){
buffer[j] = ‘\0’;
j = 0;
I don’t understand this part of the code . can i anyone help me to understand
Thank you for your great explanation, I have a question :
in the example below, how can I recognize the for loop that start from line number 5 and end in line number 8
and the same happen to while loop, I only need to recognize each loop after that the output of my tool is a new text file with the same code but before each loop a comment that show the type of the loop and other detail.
#include
using namespace std;
int main()
{
for (int a=0;a<=10;a++)
{
cout<<"hello"<<endl;
}
int a = 10;
while( a < 20 ) {
cout << "value of a: " << a << endl;
a++;
}
return 0;
}
can you explain full code
return_type main()
{
switch (varible)
begin
case num :
var= id/num
printf(var);
break;
end
return 0;
}
create code for this in c language lexical code
after compilation of the c++ program it says, there is an error while opening the file. Can anybody help me with this
Sir I need this in C++ with oop…how to do this??
how to implement lexcal analyser for if statement ?
If I want to change the C program to only have 7 keywords what do I have to change?
Where to put the other file program.txt in system?
Assume you have the following C++ source program to be analyzed by C++ compiler
lexical analyzer. Identify and list down all possible tokens and their lexemes.
#include
int Avg(int list[], int n)
( int s=0;
for(int i=0;i<n;i++)
s+=i;
return float(s)/n;
)
int main()
{ int x[]={7,6,3,1};
cout<<”the average of the numbers is ”<<Avg(x,4);
return 0;
}
Use the following table to write your answers.
Token
Matching
Constant Tokenization(Number Tokenization)